上一篇博文中,说明了怎么引进Prometheus到asp.net core项目中,因为是Demo,所以Prometheus和Grafana都是windows版本,本地执行的,生产环境上这些服务可以根据的公司的架构,放到适合的环境内,现在这些服务都支持跨平台化和容器化。并且在上篇博客中展示的是http请求的基础信息模板,本篇博客介绍自定义Prometheusr指标类型。
Prometheus有四种指标类型:Counter(计数器)、Gauge(仪表盘)、Histogram(直方图)、Summary(摘要),如果对业务的指标进行收集展示,在项目中是侵入式编程的,如果项目使用Prometheus.net进行对接Permetheus,是通过该包中的静态方法 Metrics.CreateCounter(),Metrics.CreateGauge(),Metrics.CreateSummary(),Metrics.CreateHistogram()来创建静态指标收集器,完成对业务指标收集的。
我们先来看具体Demo。
1、Counter:计数器,只增不减
先设置个业务场景:比如做一个商城,有用户注册(/register),下订单(/order),支付(/pay),发货(/ship)四个API,代码如下:
using Microsoft.AspNetCore.Mvc;
using Microsoft.Extensions.Logging;
using PrometheusSample.Models;
using PrometheusSample.Services;
using System;
using System.Threading.Tasks;
namespace PrometheusSample.Controllers
{
[ApiController]
[Route("[controller]")]
public class BusinessController : ControllerBase
{
private readonly ILogger<BusinessController> _logger;
private readonly IOrderService _orderService;
public BusinessController(ILogger<BusinessController> logger, IOrderService orderService)
{
_orderService = orderService;
_logger = logger;
}
/// <summary>
/// 注册
/// </summary>
/// <param name="username">用户名</param>
/// <returns></returns>
[HttpPost("/register")]
public async Task<IActionResult> RegisterUser([FromBody] User user)
{
try
{
_logger.LogInformation("用户注册");
var result = await _orderService.Register(user.UserName);
if (result)
{
return new JsonResult(new { Result = true });
}
else
{
return new JsonResult(new { Result = false });
}
}
catch (Exception exc)
{
_logger.LogCritical(exc, exc.Message);
return new JsonResult(new { Result = false, Message = exc.Message });
}
}
[HttpGet("/order")]
public IActionResult Order(string orderno)
{
try
{
_logger.LogInformation("下单");
return new JsonResult(new { Result = true });
}
catch (Exception exc)
{
_logger.LogCritical(exc, exc.Message);
return new JsonResult(new
{
Result = false,
Message = exc.Message
});
}
}
[HttpGet("/pay")]
public IActionResult Pay()
{
try
{
_logger.LogInformation("支付");
return new JsonResult(new { Result = true });
}
catch (Exception exc)
{
_logger.LogCritical(exc, exc.Message);
return new JsonResult(new { Result = false, Message = exc.Message });
}
}
[HttpGet("/ship")]
public IActionResult Ship()
{
try
{
_logger.LogInformation("发货");
return new JsonResult(new { Result = true });
}
catch (Exception exc)
{
_logger.LogCritical(exc, exc.Message);
return new JsonResult(new { Result = false, Message = exc.Message });
}
}
}
}
上面是基本的业务Controller,为了降低依赖,我们的业务指标收集统一到一个中间件中去收集,中间件根据请求的url,和返回的数据结果数据进行业务指标数据的收集,当然也可以引入action过滤器或MediatR等中介者模式的组件来隔离业务逻辑的开发与监控数据的采集。
本例是用中间件的方式,首先定义一个静态的指标收集器:
public class MetricsHub
{
private static Dictionary<string, Counter> _counterDictionary = new Dictionary<string, Counter>();
public Counter GetCounter(string key)
{
if (_counterDictionary.ContainsKey(key))
{
return _counterDictionary[key];
}
else
{
return null;
}
}
public void AddCounter(string key, Counter counter)
{
_counterDictionary.Add(key, counter);
}
}
定义中间件BusinessMetricsMiddleware
using Microsoft.AspNetCore.Http;
using PrometheusSample.Models;
using System.IO;
using System.Threading.Tasks;
namespace PrometheusSample.Middlewares
{
/// <summary>
/// 请求记录中间件
/// </summary>
public class BusinessMetricsMiddleware
{
private readonly RequestDelegate _next;
public BusinessMetricsMiddleware(RequestDelegate next)
{
_next = next;
}
public async Task InvokeAsync(HttpContext context, MetricsHub metricsHub)
{
var originalBody = context.Response.Body;
try
{
using (var memStream = new MemoryStream())
{
//从管理返回的Response中取出返回数据,根据返回值进行监控指标计数
context.Response.Body = memStream;
await _next(context);
memStream.Position = 0;
string responseBody = new StreamReader(memStream).ReadToEnd();
memStream.Position = 0;
await memStream.CopyToAsync(originalBody);
if (metricsHub.GetCounter(context.Request.Path) != null || metricsHub.GetGauge(context.Request.Path) != null)
{
//这里约定所有action返回值是一个APIResult类型
var result = System.Text.Json.JsonSerializer.Deserialize<APIResult>(responseBody, new System.Text.Json.JsonSerializerOptions { PropertyNameCaseInsensitive = true });
if (result != null && result.Result)
{
//获取到Counter
var counter = metricsHub.GetCounter(context.Request.Path);
if (counter != null)
{
//计数
counter.Inc();
}
}
}
}
}
finally
{
context.Response.Body = originalBody;
}
}
}
}
中间件中,只要action请求返回的Result为true,就会计数,这样做的前提条件是业务返回值有统一约定;但每个action返回不可能都一样的,如果有特例,可以用action过滤器或中介者模式组件来对应。
再看一下Starup中是怎么配置这个中间件的:
using Microsoft.AspNetCore.Builder;
using Microsoft.AspNetCore.Hosting;
using Microsoft.AspNetCore.Mvc;
using Microsoft.Extensions.Configuration;
using Microsoft.Extensions.DependencyInjection;
using Microsoft.Extensions.Hosting;
using Microsoft.Extensions.Logging;
using Microsoft.OpenApi.Models;
using Prometheus;
using PrometheusSample.Middlewares;
using PrometheusSample.Services;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
namespace PrometheusSample
{
public class Startup
{
public Startup(IConfiguration configuration)
{
Configuration = configuration;
}
public IConfiguration Configuration { get; }
public void ConfigureServices(IServiceCollection services)
{
MetricsHandle(services);
services.AddScoped<IOrderService, OrderService>();
services.AddControllers();
services.AddSwaggerGen(c =>
{
c.SwaggerDoc("v1", new OpenApiInfo { Title = "PrometheusSample", Version = "v1" });
});
}
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
app.UseSwagger();
app.UseSwaggerUI(c => c.SwaggerEndpoint("/swagger/v1/swagger.json", "PrometheusSample v1"));
}
app.UseRouting();
//http请求的中间件
app.UseHttpMetrics();
app.UseAuthorization();
//自定义业务跟踪
app.UseBusinessMetrics();
app.UseEndpoints(endpoints =>
{
//映射监控地址为 /metrics
endpoints.MapMetrics();
endpoints.MapControllers();
});
}
/// <summary>
/// 处理监控事项
/// </summary>
/// <param name="services"></param>
void MetricsHandle(IServiceCollection services)
{
var metricsHub = new MetricsHub();
//counter
metricsHub.AddCounter("/register", Metrics.CreateCounter("business_register_user", "注册用户数。"));
metricsHub.AddCounter("/order", Metrics.CreateCounter("business_order_total", "下单总数。"));
metricsHub.AddCounter("/pay", Metrics.CreateCounter("business_pay_total", "支付总数。"));
metricsHub.AddCounter("/ship", Metrics.CreateCounter("business_ship_total", "发货总数。"));
services.AddSingleton(metricsHub);
}
}
}
MetricsHandle中,我们添加了四个action,分别对应的四个计数器,这样,当这四个url有请求,并且返回值中的result=true时,就会往对应的计数器上计数。
这样数据收集好了,现在开始在Grafana中配置显示的图表了:
订单各状态总数配置:

订单各状态30秒内数量跟踪折线
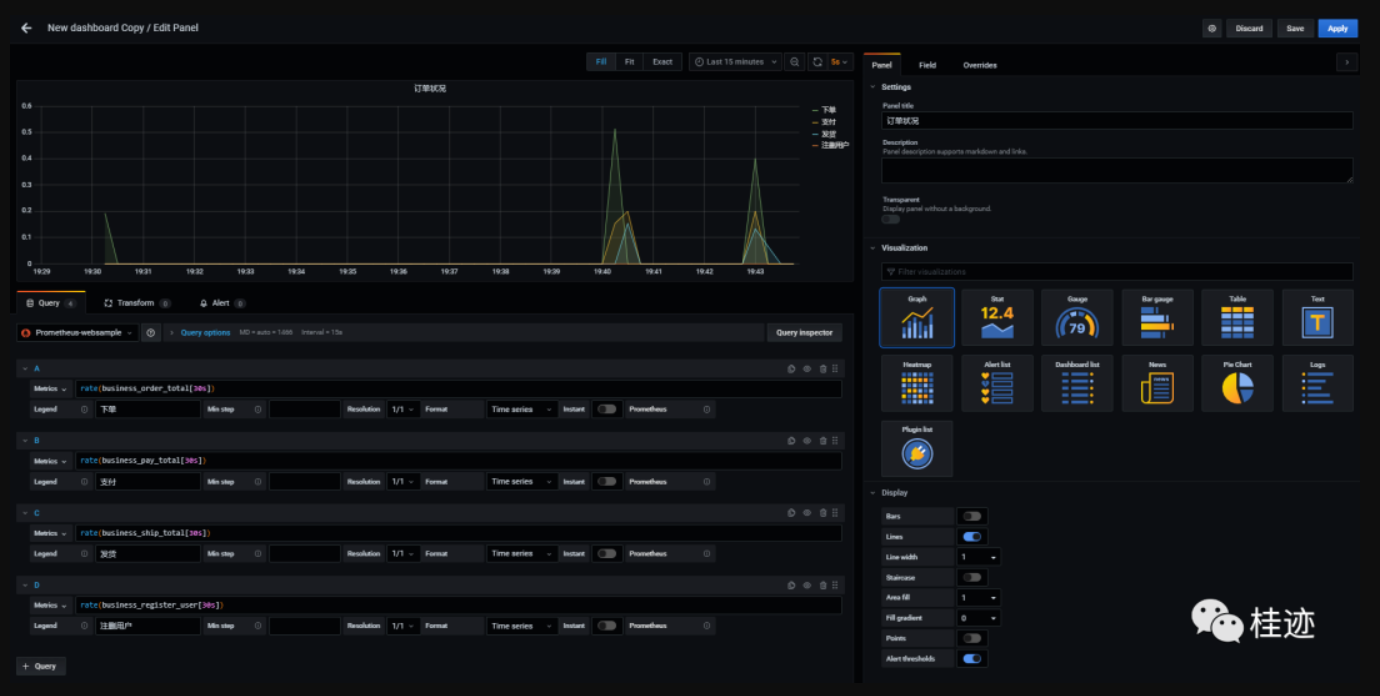
最后的运行结果是:

总结实现自定义业务计数器步骤:
1、分析业务,规划好监控跟踪指标
2、定义指标收集器
3、侵入编程(尽量在开发时分离业务实现与监控指票的收集代码)收集指标
4、开发grafana展示模板,完成展示