前面几章节中,我们都是基于 ASP.NET 空项目 模板创建的 HelloWorld 上做开发
通过这个最基本的 HelloWorld 项目,我们了解了很多知识,初窥了 ASP.NET Core,并对 ASP.NET Core 的运行机制有了一个基本的了解
MVC 模式是 Web 开发中最重要的一个模式之一,通过 MVC,我们可以将控制器、模型和视图区分开来
ASP.NET Core 同样支持 MVC 模式,而且是通过中间件的形式来支持 MVC 模式的开发
MVC 中间件
一般情况下,ASP.NET Core 2.1 内置并下载了 Microsoft.AspNetCore.Mvc
程序集
所以我们并不需要使用 NuGet
来做一些额外的安装
我们只需要给我们的应用程序中注册 Microsoft.AspNetCore.Mvc
中间件即可
配置 MVC 中间件
我们需要将 ASP.NET Core MVC 所需的所有服务注册到运行时中
我们在 Startup
类中的 ConfigureServices()
方法中执行此操作
注册完毕后,我们将添加一个简单的控制器,然后使用控制器做一些简单的输出
- 我们先在跟目录下创建一个目录
Controllers
目录,用于存放所有的控制器
右键点击 HelloWorld 项目,然后选择 添加 -> 新建文件夹,并把文件夹命名为 Controllers
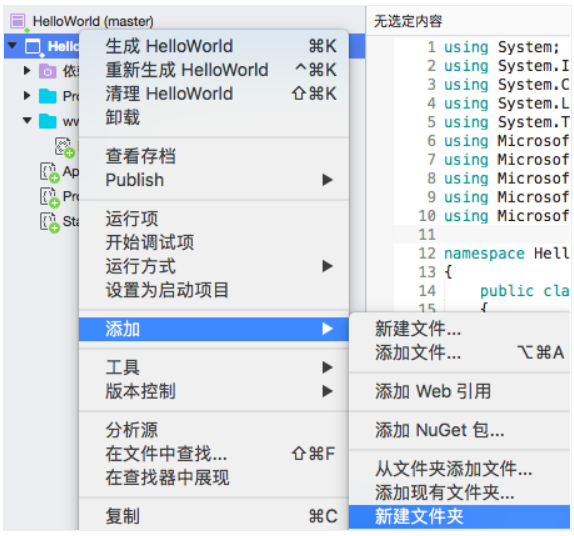
- 添加完成后 解决方案资源管理器 中显示如下
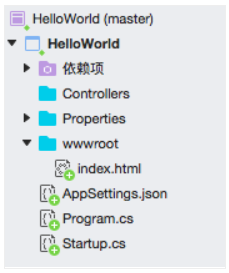
- 右键点击
Controllers
目录,然后选择 添加 -> 新建文件 打开新建文件对话框如果你的电脑是 Windows ,则是 添加 -> 新建项

- 在新建文件对话框中,选中左边的 General,然后选中右边的 空类如果你的电脑是 Windows ,则是先选中 ASP.NET Core 下的 代码 , 然后选中 类
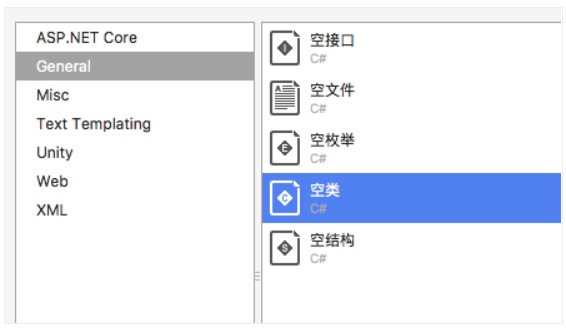
- 在名称中输入 HomeController,然后点击右下角的 新建 按钮,创建一个 HomeController.cs 文件如果你的电脑是 Windows ,则是点击右下角的 新建 按钮

- 添加完成后 解决方案资源管理器 中显示如下
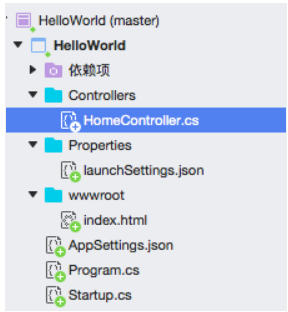
- 同时可以看到
HomeController.cs
中的内容如下
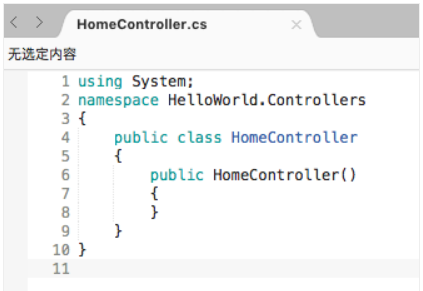
using System;
namespace HelloWorld.Controllers
{
public class HomeController
{
public HomeController()
{
}
}
}
- 接下来我们将设置
HomeController
类为我们的默认控制器,也就是访问/
时默认使用HomeController
来处理 - 修改
HomeController.cs
文件,为类HomeController
类添加一个Index()
方法
public string Index()
{
return "你好,世界! 此消息来自 HomeController...";
}
- 文件全部内容如下
using System;
namespace HelloWorld.Controllers
{
public class HomeController
{
public HomeController()
{
}
public string Index()
{
return "你好,世界! 此消息来自 HomeController...";
}
}
}
- 保存 **HomeController.cs
文件,重新启动应用并刷新浏览器,显示的仍然是
index.html` 中的内容
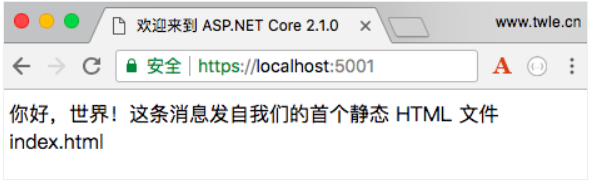
- 现在,我们删除
wwwroot
目录下的index.html
文件右键点击index.html
文件,然后选择 删除,在弹出的对话框中点击 删除 按钮 - 然后我们回到
Startup.cs
文件中,在Configure()
方法中的app.UseFileServer();
语句后面添加一条语句app.UseMvcWithDefaultRoute();Startup.cs
文件全部代码如下
using System;
using System.IO;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using Microsoft.AspNetCore.Builder;
using Microsoft.AspNetCore.Hosting;
using Microsoft.AspNetCore.Http;
using Microsoft.Extensions.DependencyInjection;
using Microsoft.Extensions.Configuration;
namespace HelloWorld
{
public class Startup
{
public Startup()
{
var builder = new ConfigurationBuilder()
.SetBasePath(Directory.GetCurrentDirectory())
.AddJsonFile("AppSettings.json");
Configuration = builder.Build();
}
public IConfiguration Configuration { get; set; }
// This method gets called by the runtime. Use this method to add services to the container.
// For more information on how to configure your application, visit https://go.microsoft.com/fwlink/?LinkID=398940
public void ConfigureServices(IServiceCollection services)
{
}
// This method gets called by the runtime. Use this method to configure the HTTP request pipeline.
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
app.UseFileServer();
app.UseMvcWithDefaultRoute();
/*
app.Run(async (context) =>
{
var msg = Configuration["message"];
await context.Response.WriteAsync(msg);
});
*/
}
}
}
- 保存 Startup.cs 文件,重新启动应用,会发现启动失败,出错如下
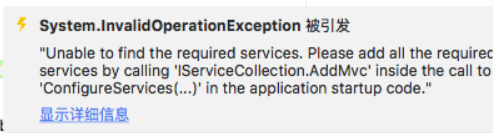
System.InvalidOperationException: "Unable to find the required services. Please add all the required services by calling 'IServiceCollection.AddMvc' inside the call to 'ConfigureServices(...)' in the application startup code."
- 意思是 ASP.NET Core 没有找到必须的 Mvc 服务ASP.NET 核心框架本身由具有非常专注的责任的不同小型组件组成例如,有一个组件必须定位和实例化控制器,但该组件需要位于 ASP.NET Core MVC 的服务集合中才能正常运行
注册 MVC 服务
为了在 ASP.NET Core 中使用 MVC 模式,我们必须在 Startup
类中的 ConfigureServices
方法中添加 AddMvc
服务
public void ConfigureServices(IServiceCollection services)
{
services.AddMvc();
}
添加成功后,完整的 Startup.cs
文件如下
using System;
using System.IO;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using Microsoft.AspNetCore.Builder;
using Microsoft.AspNetCore.Hosting;
using Microsoft.AspNetCore.Http;
using Microsoft.Extensions.DependencyInjection;
using Microsoft.Extensions.Configuration;
namespace HelloWorld
{
public class Startup
{
public Startup()
{
var builder = new ConfigurationBuilder()
.SetBasePath(Directory.GetCurrentDirectory())
.AddJsonFile("AppSettings.json");
Configuration = builder.Build();
}
public IConfiguration Configuration { get; set; }
// This method gets called by the runtime. Use this method to add services to the container.
// For more information on how to configure your application, visit https://go.microsoft.com/fwlink/?LinkID=398940
public void ConfigureServices(IServiceCollection services)
{
services.AddMvc();
}
// This method gets called by the runtime. Use this method to configure the HTTP request pipeline.
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
app.UseFileServer();
app.UseMvcWithDefaultRoute();
/*
app.Run(async (context) =>
{
var msg = Configuration["message"];
await context.Response.WriteAsync(msg);
});
*/
}
}
}
保存 Startup.cs 文件,重新启动应用,刷新浏览器,终于可以看到结果了
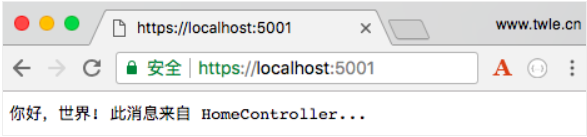