上一章节我们终于迁移完了 Identity 的数据,也创建了用户表,现在,磨拳擦掌,是时候把注册功能给完善了。当然了,要实现登录功能得先有个用户,添加用户的方法很多,但是,我们还是先实现一个用户注册功能吧
本章节我们先来实现一个用户注册的功能,注册用户的 URL 为 /Account/Signup
,也就是添加一个 AccountController
控制器,然后再实现一个 Signup
的动作方法,这也是为了以后匹配登录动作的 URL /Account/Login
- 首先在
Controllers
目录下新建一个AccountController.cs
文件,创建方法和当初创建AbountController.cs
一样
创建完成后目录结构如下
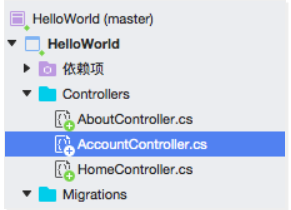
而 AccountController.cs
中的默认内容如下
using System;
namespace HelloWorld.Controllers
{
public class AccountController
{
public AccountController()
{
}
}
}
- 修改
AccountController.cs
引入命名空间Microsoft.AspNetCore.Mvc
,并让AccountController
继承自该命名空间下的Controller
using System;
using Microsoft.AspNetCore.Mvc;
namespace HelloWorld.Controllers
{
public class AccountController: Controller
{
public AccountController()
{
}
}
}
- 然后在
AccountController
类中创建Register
用来显示注册表单
[HttpGet]
public ViewResult Register() {
return View();
}
- 接下来在
Views
目录下创建目录Account
,并在Views/Account
目录下新建普通视图Signup.cshtml
创建完成后目录结构如下
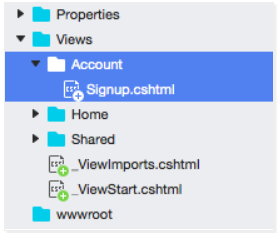
Signup.cshtml
中的默认内容如下
@*
For more information on enabling MVC for empty projects, visit https://go.microsoft.com/fwlink/?LinkID=397860
*@
@{
}
- 再我们继续修改
Signup.cshtml
之前,我们先要确认一件事,就是注册的时候需要输入多少内容,我们回到 SQLite Studio,看看我们的用户表有哪些字段
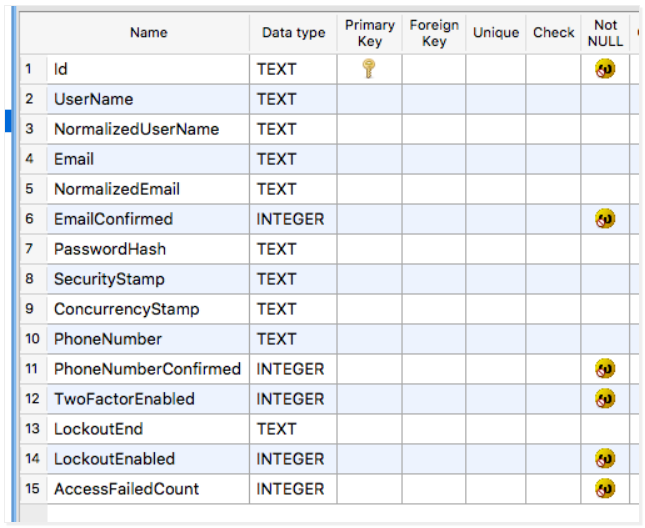
可以看到几个熟悉的身影 UserName
、Email
和 PasswordHash
,也就是说我们既可以使用用户名作为唯一标识,也可以使用邮箱作为唯一标识,两个都可以使用,也可以只用一个,我们这里就使用用户名吧
于是,我们的注册表单就要用户输入三项内容 UserName
和 Password
,再加上确认命名 ConfirmPassword
- 好的,我们可以建模了,我们先创建一个模型
RegisterViewModel
来收集用户提交的信息,我们把这个模型放在AccountController.cs
文件中
public class RegisterViewModel {
[Required, MaxLength(64)]
public string Username { get; set; }
[Required, DataType(DataType.Password)]
public string Password { get; set; }
[DataType(DataType.Password), Compare(nameof(Password))]
public string ConfirmPassword { get; set; }
}
我们使用 C# 特性来帮助我们验证数据,当然多了几个新的面孔,比如 DataType
和 Compare
DataType
用于设置渲染成 HTML 时所使用的表单类型,再这里,就是type="password"
Compare
用于验证数据时指定和Password
要一样
当然了,为了使用这些 C# 特性,还需要引入命名空间 System.ComponentModel.DataAnnotations
- 修改完成后
AccountController.cs
中的内容如下
using System;
using Microsoft.AspNetCore.Mvc;
using System.ComponentModel.DataAnnotations;
namespace HelloWorld.Controllers
{
public class AccountController : Controller
{
public AccountController()
{
}
[HttpGet]
public ViewResult Signup()
{
return View();
}
}
public class RegisterViewModel
{
[Required, MaxLength(64)]
public string Username { get; set; }
[Required, DataType(DataType.Password)]
public string Password { get; set; }
[DataType(DataType.Password), Compare(nameof(Password))]
public string ConfirmPassword { get; set; }
}
}
- 好了,有了这些数据我们就可以修改
Signup.cshtml
了
@model RegisterViewModel
@{
ViewBag.Title = "注册用户";
}
<h1>注册用户</h1>
<form asp-action="Signup" method="post">
<div asp-validation-summary = "ModelOnly"></div>
<div>
<label asp-for="Username">用 户 名</label>
<input asp-for="Username" />
<span asp-validation-for="Username"></span>
</div>
<div>
<label asp-for="Password">密 码</label>
<input asp-for="Password" />
<span asp-validation-for="Password"></span>
</div>
<div>
<label asp-for="ConfirmPassword">确认密码</label>
<input asp-for="ConfirmPassword" />
<span asp-validation-for="ConfirmPassword"></span>
</div>
<div>
<input type="submit" value="注册" />
</div>
</form>
除了 <div asp-validation-summary = "ModelOnly"></div>
这个应该没啥新鲜的内容了
asp-validation-summary
是一个 HTML 标签助手,用于输出所有的验证的错误信息的概要信息
而 ModelOnly
是类 ValidationSummary
的一个成员,意思是只输出模型验证的错误信息
- 因为我们在注册表单中指定了使用 HTTP POST 方法把数据提交到
Signup
动作方法上,所以我们需要在AccountController
里重载Signup
动作方法
[HttpPost]
public IActionResult Signup(RegisterViewModel model)
{
return View();
}
这个动作方法将收到一个 RegisterViewModel
然后返回一个 IActionResult
在这个方法中需要与 Identity 框架进行交互,以确保用户有效,告诉 Identity 框架创建该用户
但这涉及到的内容实在太多,我们留给下一章节来填充,本章节我们留空就好
- 修改完成后,
AccountController.cs
的全部内容如下
using System;
using Microsoft.AspNetCore.Mvc;
using System.ComponentModel.DataAnnotations;
namespace HelloWorld.Controllers
{
public class AccountController : Controller
{
public AccountController()
{
}
[HttpGet]
public ViewResult Signup()
{
return View();
}
[HttpPost]
public IActionResult Signup(RegisterViewModel model)
{
return View();
}
}
public class RegisterViewModel
{
[Required, MaxLength(64)]
public string Username { get; set; }
[Required, DataType(DataType.Password)]
public string Password { get; set; }
[DataType(DataType.Password), Compare(nameof(Password))]
public string ConfirmPassword { get; set; }
}
}
- 好了,我们回到
Index.cshtml
添加一个 注册新用户 的链接指向刚刚创建的Account/Signup
地址
@model HomePageViewModel
@{
ViewBag.Title = "职工列表";
}
<style>
body {margin:10px auto;text-align:center}
table {
margin:0 auto;
width:90%
}
table, th, td {
border:1px solid #eee;
border-collapse:collapse;
border-spacing:0;
padding:5px;
text-align:center
}
.txt-left {
text-align:left;
}
</style>
<h1>职工列表</h1>
<table>
<tr>
<td>ID</td>
<td>姓名</td>
<td class="txt-left">操作</td>
</tr>
@foreach (var employee in Model.Employees)
{
<tr>
<td>@employee.ID</td>
<td>@employee.Name</td>
<td class="txt-left"><a asp-action="Detail" asp-route-Id="@employee.ID">详情</a> <a asp-controller="Home" asp-action="Edit"
asp-route-id="@employee.ID">编辑</a></td>
</tr>
}
</table>
<br/>
<p><a asp-action="Create" >新增员工</a></p>
<hr/>
<p><a asp-controller="Account" asp-action="Signup">注册新用户</a></p>
asp-controller="Account"
用户指定链接的控制器名为 Account
好了,保存所有的代码,然后重启应用程序,访问首页,显示如下
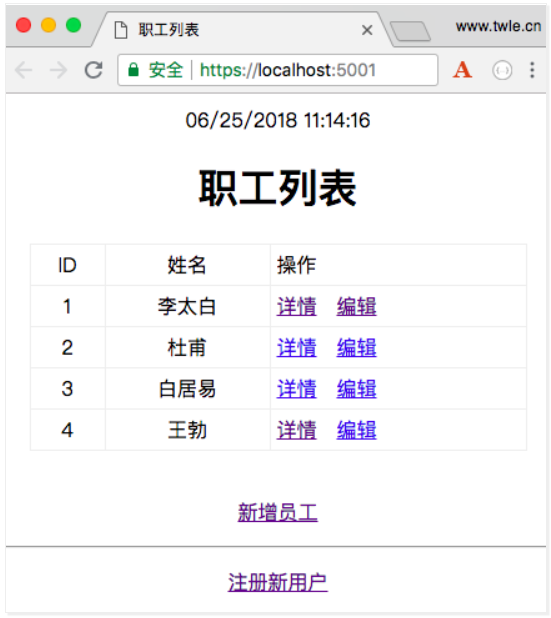
点击 注册新用户 显示如下
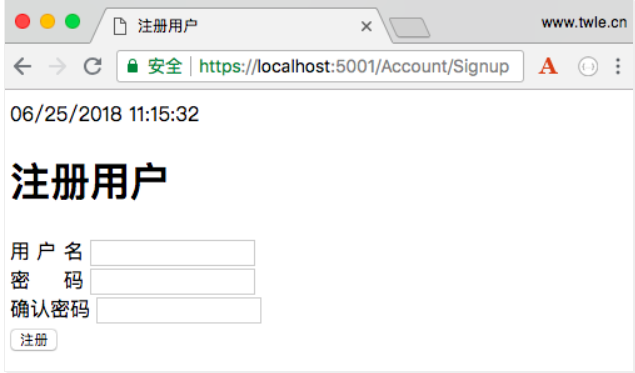
如果我们不输入任何数据,直接点击 注册 按钮,则显示如下
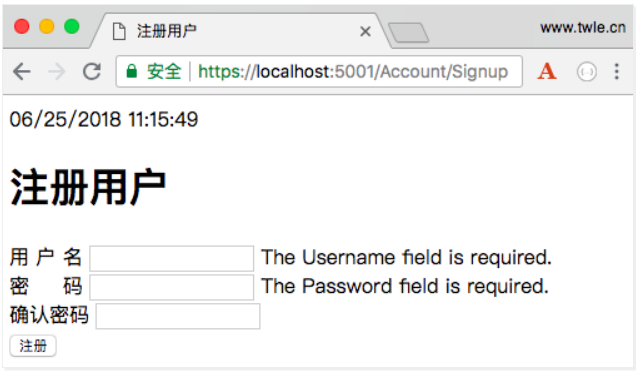
如果输入完整的数据,点击 注册 则显示如下
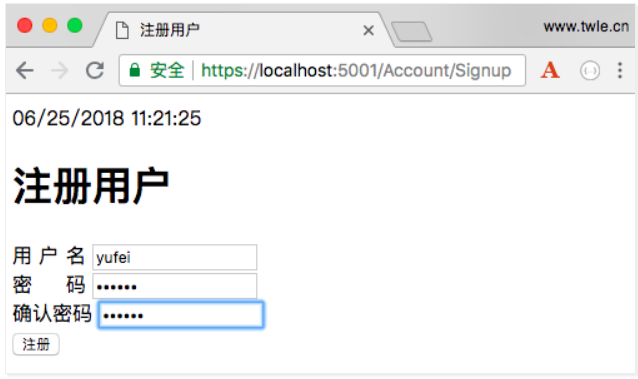
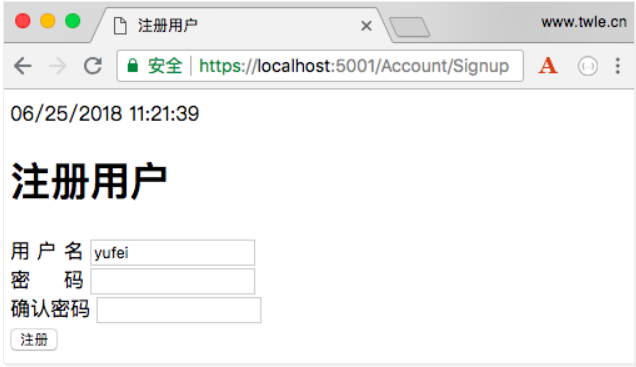